In this tutorial, I will show you how to write a Clickbot for the famous Cookie Clicker game by orteil. With this little hack, you can click as fast as your hardware allows to generate endless cookies. We will use python3 and a module called selenium. It is mostly used for testing websites but also the perfect tool for automating tasks like rapid clicking on a cookie.
How does selenium work?
Selenium is basically a testing framework for websites and compatible with Windows, Mac and Linux. It uses a Webdriver to access the installed Browser to simulate user interaction. In this tutorial, I will use the chromedriver for Google Chrome Browser, but you can use any driver by modifying the code. In Firefox’s case it’s called Geckodriver and can be download from the official Github Page.
Cookie Clickbot in action
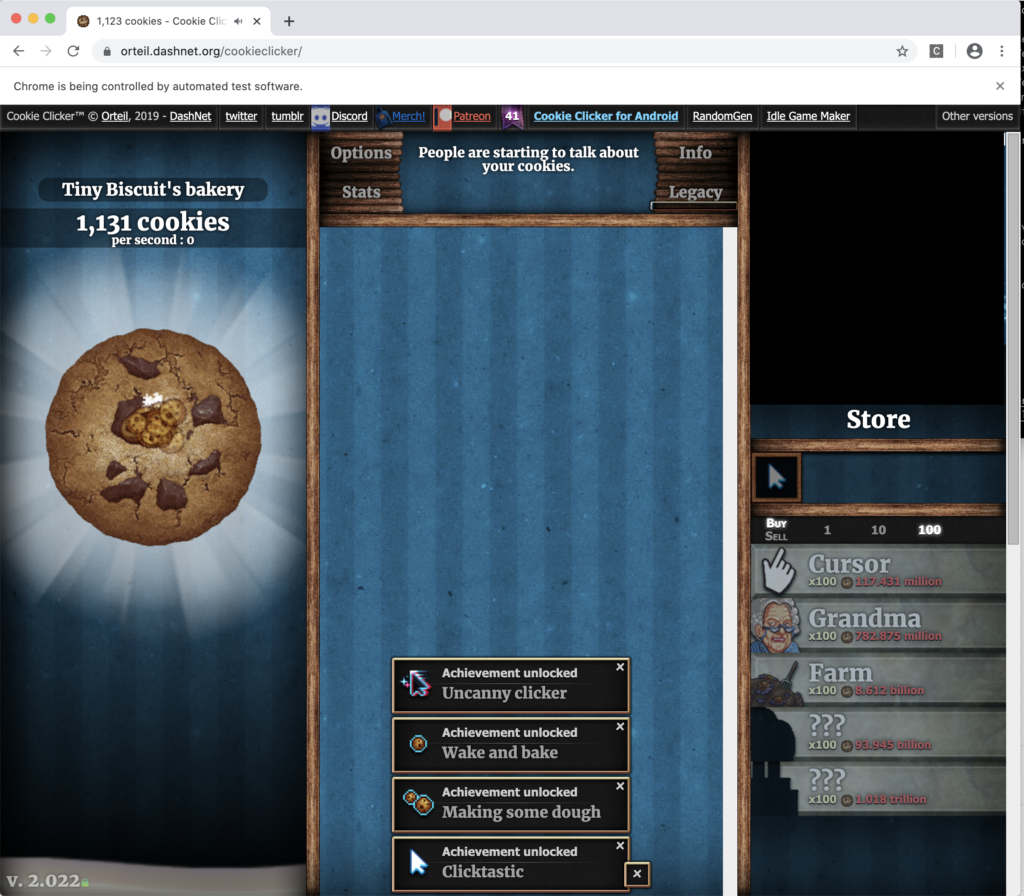
Let’s look at the python code
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
import time, sys
class cookieBot():
def __init__(self):
self.browser = webdriver.Chrome(sys.argv[1])
self.browser.get("https://orteil.dashnet.org/cookieclicker/")
WebDriverWait(self.browser, 4).until(EC.presence_of_element_located((By.ID, 'bigCookie')))
In the beginning, we import WebDriver, which will open and control the browser. With WebDriverWait and EC, we can wait until expected conditions are met, for example a specific element has loaded. We use By to easily identify elements in the DOM of the website and time to wait for a specific duration in seconds.
In the next step, we create the class cookieBot with a constructor opening the Cookie Clicker Website and wait until the page has fully loaded. If you want to use Firefox instead of Chrome, you can modify self.browser to:
self.browser = webdriver.Firefox(sys.argv[1])
Keep in mind that you need the corresponding GeckoDriver for your Firefox version. You can download the latest one from the official Github Page here.
The next line is opening Cookie Clickers Website in the browser. After that we want to wait until the clickable Cookie has loaded, otherwise the program will throw an error on access. This is done by WebDriverWait with the expected condition that an element with id=bigCookie has already loaded.
Performing the automated click
Now we declare the class function clickCookie(). It will be called in an endless loop later to perform the click on the Cookie to generate income. Therefore we use the selenium built-in function find_element_by_id() to identify the Cookie. In the next instruction we click on it.
def clickCookie(self):
cookie = self.browser.find_element_by_id('bigCookie')
cookie.click()
Program flow
At first we create an object of the class cookieBot. This will open the browser and open Cookie Clicker’s page. After that we wait for one second. Therefore the browser has time to load all remaining resources to build the page. In the end we execute an infinite loop clicking on the cookie.
bot = cookieBot()
time.sleep(1)
while(True):
bot.clickCookie()
You can clone the GitHub repository containing the whole code with the following command:
git clone https://github.com/jreinhardt2/CookieClicker.git
Execution
After cloning the repository and changing into root directory, you can execute the Clickbot with following command:
python3 clicker.py "path_to_chromedriver"
The repository contains the corresponding driver for Windows, Mac and Linux. It’s important to specify the path to the file. You can either use an absolute path like:
python3 clicker.py "/Users/Julius/Documents/CookieClicker/chromedriver_mac"
or a relative path like:
python3 clicker.py "./chromedriver_mac"
Remember to not forget ./ at the beginning of the relative path!
Clickbot code in python
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
import time, sys
if len(sys.argv) < 2:
print('Usage: python clicker.py "path_to_chromedriver" || In same directory add ./ before chromedriver')
sys.exit(-1)
class cookieBot():
def __init__(self):
self.browser = webdriver.Chrome(sys.argv[1])
self.browser.get("https://orteil.dashnet.org/cookieclicker/")
WebDriverWait(self.browser, 4).until(EC.presence_of_element_located((By.ID, 'bigCookie')))
def clickCookie(self):
cookie = self.browser.find_element_by_id('bigCookie')
cookie.click()
bot = cookieBot()
time.sleep(1)
while(True):
bot.clickCookie()
Conclusion
As you can see it is possible with selenium to use very little code to write a Clickbot for Cookie Clicker. You can apply this tutorial to every element on a desired website you want to click.
If you are interested in how I started this blog you can check it out here or if you always wanted to know how to decrypt Nodejs TLS traffic you can check here.